📜 [專欄新文章] Gas Efficient Card Drawing in Solidity
✍️ Ping Chen
📥 歡迎投稿: https://medium.com/taipei-ethereum-meetup #徵技術分享文 #使用心得 #教學文 #medium
Assign random numbers as the index of newly minted NFTs
Scenario
The fun of generative art NFT projects depends on randomness. The industry standard is “blind box”, where both the images’ serial number and the NFTs’ index are predetermined but will be shifted randomly when the selling period ends. (They call it “reveal”) This approach effectively solves the randomness issue. However, it also requires buyers to wait until the campaign terminates. What if buyers want to know the exact card right away? We’ll need a reliable onchain card drawing solution.
The creator of Astrogator🐊 isn’t a fan of blind boxes; instead, it thinks unpacking cards right after purchase is more interesting.
Spec
When initializing this NFT contract, the creator will determine the total supply of it. And there will be an iterable function that is randomly picking a number from the remaining pool. The number must be in range and must not collide with any existing ones.
Our top priority is accessibility/gas efficiency. Given that gas cost on Ethereum is damn high nowadays, we need an elegant algorithm to control gas expanse at an acceptable range.
Achieving robust randomness isn’t the primary goal here. We assume there’s no strong financial incentive to cheat, so the RNG isn’t specified. Implementers can bring their own source of randomness that they think is good enough.
Implementation
Overview
The implementation is pretty short and straightforward. Imagine there’s an array that contains all remaining(unsold) cards. When drawIndex() is called, it generates a (uniform) random seed to draw a card from the array, shortens the array, and returns the selected card.
Algorithm
Drawing X cards from a deck with the same X amount of cards is equal to shuffling the deck and dealing them sequentially. It’s not a surprise that our algorithm is similar to random shuffling, and the only difference is turning that classic algo into an interactive version.
A typical random shuffle looks like this: for an array with N elements, you randomly pick a number i in (0,N), swap array[0] and array[i], then choose another number i in (1,N), swap array[1] and array[i], and so on. Eventually, you’ll get a mathematically random array in O(N) time.
So, the concept of our random card dealing is the same. When a user mints a new card, the smart contract picks a number in the array as NFT index, then grabs a number from the tail to fill the vacancy, in order to keep the array continuous.
Tweak
Furthermore, as long as the space of the NFT index is known, we don’t need to declare/initialize an array(which is super gas-intensive). Instead, assume there’s such an array that the n-th element is n, we don’t actually initialize it (so it is an array only contains “0”) until the rule is broken.
For the convenience of explanation, let’s call that mapping cache. If cache[i] is empty, it should be interpreted as i instead of 0. On the other hand, when a number is chosen and used, we’ll need to fill it up with another unused number. An intuitive method is to pick a number from the end of the array, since the length of the array is going to decrease by 1.
By doing so, the gas cost in the worst-case scenario is bound to be constant.
Performance and limitation
Comparing with the normal ascending index NFT minting, our random NFT implementation requires two extra SSTORE and one extra SLOAD, which cost 12600 ~ 27600 (5000+20000+2600) excess gas per token minted.
Theoretically, any instantly generated onchain random number is vulnerable. We can restrict contract interaction to mitigate risk. The mitigation is far from perfect, but it is the tradeoff that we have to accept.
ping.eth
Gas Efficient Card Drawing in Solidity was originally published in Taipei Ethereum Meetup on Medium, where people are continuing the conversation by highlighting and responding to this story.
👏 歡迎轉載分享鼓掌
同時也有47部Youtube影片,追蹤數超過22萬的網紅Zermatt Neo,也在其Youtube影片中提到,For this episode, we headed back to Neo’s Kitchen to attempt a LEVEL 99 SPICY MALA WONTON CHALLENGE! EB Food is organising a timed wonton challenge wi...
「a number of an amount of」的推薦目錄:
- 關於a number of an amount of 在 Taipei Ethereum Meetup Facebook 的最佳貼文
- 關於a number of an amount of 在 Eric Fan 范健文 Facebook 的精選貼文
- 關於a number of an amount of 在 TradingwithRayner Facebook 的精選貼文
- 關於a number of an amount of 在 Zermatt Neo Youtube 的最佳貼文
- 關於a number of an amount of 在 Zermatt Neo Youtube 的最讚貼文
- 關於a number of an amount of 在 Crappy Blogger Youtube 的最佳貼文
- 關於a number of an amount of 在 常春藤英語- 【#多益小教室】 多益考試中描述數量的片語很多 的評價
- 關於a number of an amount of 在 amount, number quantity的評價和優惠,PINTEREST 的評價
- 關於a number of an amount of 在 The amount of VS The number of, etc - English StackExchange 的評價
- 關於a number of an amount of 在 Amount vs Number | Ask Linda! | English Grammar - YouTube 的評價
- 關於a number of an amount of 在 Amount, quantity or number? - Pinterest 的評價
- 關於a number of an amount of 在 How to generate a random number with the amount of digits ... 的評價
a number of an amount of 在 Eric Fan 范健文 Facebook 的精選貼文
The difference between APR and APY
A lot of people here are staking their crypto, so I assume most of you have encountered these terms before, but a lot of people don't really understand the difference between the two. As such I thought I would explain the difference as simply as possible.
**APR = Annual Percentage Rate**
This is the amount you can approximately expect the initial amount of money to get in interest over one year. For example, if you staked $1000 worth of ETH and got an APR of 6%, you would expect to have about $1,060 by the end of the year:
$1000 x (1.06) = $1060
However, this is assuming that you only get one payment at the end of the year of $60 for a year's worth of interest, and we all know that by staking crypto, you get payments more often than that. Some get interest payments every week, or even every day. Now let's assume you receive interest payments daily. The amount of interest you get every day is the APR divided by 365, since there are 365 days in a year. So your daily interest rate is:
6%/365 days = 0.01643836% every day in interest
So after one day, you will have:
$1000 x (1 + 0.06/365) = $1,000.16438
Now, on the second day, it will give you the same 0.01643836% in daily interest, but it won't use the $1000 you put in initially, it will give you interest based on how much you currently have staked, which is now $1,000.16438 after one day. So after you get your interest payment on the second day, you will have:
$1,000.16438 x (1 + 0.06/365) = $1,000.32879
It will then use the number you have after two days to calculate the interest you will receive for the third day. Notice how every day, the amount of money you have is increasing, and as a result the amount of money you receive in interest increases every day. This is called *compound interest*, and that's where APY comes in.
**APY = Annual Percentage Yield**
This is the amount of interest you receive in a year taking into account compound interest. In other words, this is the how much interest you will receive taking into account you will be getting payments throughout the year. So if you have an APR like we said of 6%, and you receive interest payments daily, your APY is calculated as such:
APY = (1 + 0.06/365)^365 - 1 = 6.18313106779%
So in one year, you're actually getting 6.18313106779% in interest, not just the 6% that the APR said you were getting. So after one year you would have:
$1000 x (1.0618313106779) = $1,061.83
**To Sum Up**
Amount you will have after one year according to APR:
$1060
Amount you will have after one year according to APY:
$1,061.83
As you can see the numbers are not the same. APY just gives you a more accurate indicator as to how much you can expect to receive in interest by the end of the year.
**So which is better: 6% APR or 6% APY?**
If you noticed, the percentage I calculated in APY is higher than the percent APR said you would be getting:
6% APR = 6.18313106779% APY (with daily payments)
So, if you have the option to choose between 6% APR and 6% APY with the same frequency of payments in a year (in this case daily payments), always choose 6% APR, since that comes out to be higher than 6% APY.
Source: Reddit.
a number of an amount of 在 TradingwithRayner Facebook 的精選貼文
𝗪𝗵𝘆 𝘆𝗼𝘂𝗿 𝘀𝘁𝗼𝗽 𝗹𝗼𝘀𝘀 𝗮𝗹𝘄𝗮𝘆𝘀 𝗴𝗲𝘁𝘀 𝗵𝘂𝗻𝘁𝗲𝗱 𝗮𝗻𝗱 𝗵𝗼𝘄 𝘆𝗼𝘂 𝗰𝗮𝗻 𝗮𝘃𝗼𝗶𝗱 𝗶𝘁
Imagine…
You manage a hedge fund and want to buy 1 million shares of ABC stock. You know support is at $100 and ABC is currently trading at $110.
Now if you were to buy ABC stock right now, you’ll likely push the price higher and get filled at an average price of $115 — that’s $5 higher than the current price.
So what do you do?
Since you know $100 is an area of support, chances are, there will be a cluster of stop loss underneath it (from traders who are long ABC stock).
So, if you could push the price lower to trigger these stops, there would be a flood of sell orders hitting the market (as buyers will exit their losing positions).
With the amount of selling pressure coming in, you could buy your 1 million shares of ABC stock from these traders which gives you a better average price.
In other words, if an institution wants to long the markets with minimal slippage, they tend to place a sell order to trigger nearby stop losses. This allows them to buy from traders cutting their losses, which offers them a more favourable entry price.
Go look at your charts and you’ll often see the market taking out the lows of support, only to trade higher subsequently.
Now you’re probably wondering:
“So how do I avoid it?”
Simple.
Set your stop loss a distance away from support to give it some buffer so your stop loss doesn’t get eaten too easily.
Here’s how…
- Identify the lows of support
- Find the current Average True Range (ATR) value and subtract 1 ATR from the lows of support
The idea is to define the current market’s volatility and then subtract it from the lows of support.
This way, you are giving your stop loss a buffer that’s based on the volatility of the markets (and not just some random number).
Pro Tip:
If you want a tighter stop loss, you can reduce your ATR multiple, like having 0.5 ATR instead of 1.
a number of an amount of 在 Zermatt Neo Youtube 的最佳貼文
For this episode, we headed back to Neo’s Kitchen to attempt a LEVEL 99 SPICY MALA WONTON CHALLENGE! EB Food is organising a timed wonton challenge with the launch of their new product, EB Shrimp Wonton with Mala Sauce. Eating the greatest number of EB wontons in 5 minutes will net you a cash prize of $2000, with further cash prizes for 2nd and 3rd place. Full details listed below:
The EB Shrimp Wonton with Mala Sauce came neatly packed in a pleasantly-enough designed box that really undersold the potency of the Mala sauce. They were simple to cook - boil the 12 wontons for 5 minutes, warm up the Mala sauce in hot water and mix them all together. The Mala sauce was a menacing shade of red glistening with Mala oil radiating that signature Mala aroma. The wontons themselves respectably plumped up considerably during cooking. Each had a whole shrimp in them. When mixed together, each wonton ended up adopting an even shade of red with small clumps of Mala sauce noticeable throughout.
I attempted this challenge with Mervin (there is a buddy requirement). From the number of wontons we prepared versus the number consumed, it was clear that we underestimated this challenge. We thought it would be a speed challenge, but it ended up being a spice challenge due to our low spice tolerance and the unexpected heat of the Mala sauce. Out of the 100+ wontons we cooked, we only ate 48 between us within the 5 minutes limit.
Note that our attempt does not count for the official challenge, so please do find a buddy (preferably someone who loves Mala) and win yourself $2000! The wontons are also Halal, so Muslim viewers can join as well!
Follow these easy steps to qualify for the challenge:
1. Like and follow @ebfood.sg on both their Facebook and Instagram accounts
2. Complete the T&C Google form before joining the challenge: https://forms.gle/phDZn6q3RZ77gaYS6
3. Film and upload your challenge video on both your Instagram accounts:
- Tag @ebfood.sg and your buddy's account
- Hashtag #EBFrozenFood #BeatMAloveforLA #malasg
- Indicate the total amount of Mala Wonton consumed
- Set both accounts public
4. Send the original video with 5 minutes duration to: marketing@ebfood.sg
*Your full video should include the process of pouring and mixing all the Mala sauce given in the packaging.*
Cash Prizes:
1st Prize: $2000
2nd Prize: $1000
3rd Prize: $500
Winners will be contacted by email on 23 June 2021.
Reminder: In order to qualify for the challenge, do read through all T&Cs and rules carefully from the Google form.
Connect with us!
Facebook - https://www.facebook.com/zermattneofls
Instagram - http://instagram.com/zermattneo
http://instagram.com/teegongborpi
Use code ZERMATT for 58% off ALL Myprotein products.
For those that are interested in doing ZenyumClear™️ Aligners:
https://bit.ly/zermattneo-yt
Use code ZERMATT100 for special discount!
Hair Sponsor - Toliv Salon
5 Purvis Street, #01-03, Singapore
https://www.facebook.com/tolivboutique

a number of an amount of 在 Zermatt Neo Youtube 的最讚貼文
In this episode, we went down to Sinar Pagi Nasi Padang at Geylang Serai to destroy a 4KG Nasi Padang Challenge! Sinar Pagi Nasi Padang is a famous Nasi Padang stall that offers up a host of delicious Malay cuisine including their signature BBQ Chicken and Beef Rendang.
Nasi Padang is similar to the Chinese-style economic rice stalls common across Singapore, where you get a plate of rice and add on as much sides, ala carte, as you like. Naturally, for Nasi Padang, the sides are heavily Malay-influenced with many types of curries and spiced meat and vegetables. Most of the food would be towards the spicy side.
Unfortunately, we arrived rather close to closing time and they only had a limited number of options left. We ordered them all for this challenge. The staff very competently plated the massive amount of food beautifully onto the plate. To finish, she topped the 6 portions of rice with different types of chilli pastes and coconut atop a banana leaf, resulting in a 4KG plate of Nasi Padang.
The standout dishes would be the beef tripe curry and beef rendang. The beef tripe was extremely tender and the sauce itself was on the salty side, going fantastically with rice. The beef rendang was similarly tender, easily pulled apart with a fork. It had the classic deep spice flavour that you expect from rendangs while not being overwhelming.
This was a reasonably straightforward challenge (other than the accident at the end) that showcased my newfound spice tolerance and also helped to push it further. As mentioned earlier, we came too late and missed many of the “better” dishes Sinar Pagi offers, but it was still an enjoyable meal.
Visit Sinar Pagi Nasi Padang for one of the most highly rated Nasi Padangs in Singapore!
Sinar Pagi Nasi Padang location:
1 Geylang Serai #02-137
Singapore 402001
Connect with us!
Facebook - https://www.facebook.com/zermattneofls
Instagram - http://instagram.com/zermattneo
http://instagram.com/teegongborpi
Use code ZERMATT for 58% off ALL Myprotein products.
For those that are interested in doing Invisible Braces:
https://bit.ly/zermattneo-yt
Use code ZERMATT100 for special discount!
Hair Sponsor - Toliv Salon
5 Purvis Street, #01-03, Singapore
https://www.facebook.com/tolivboutique
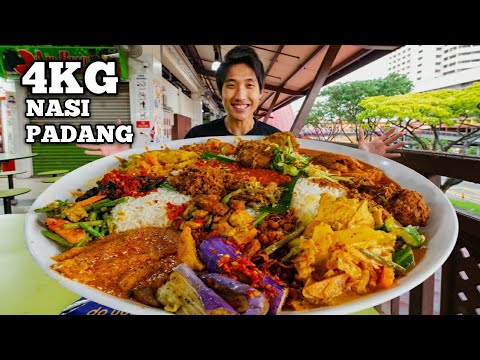
a number of an amount of 在 Crappy Blogger Youtube 的最佳貼文
For more follow at
Facebook: https://www.facebook.com/crappyblogger/
Instagram: https://www.instagram.com/crappyblogger_/
Blog: www.crappyblogger.com
Psyched Blog: https://www.crappyblogger.com/search/label/Psyched
Apparently, stress and boredom cause you to indulge in your bad habits. I found it to be true as I always shop whenever I am stressed out.
It is a hell of habit as shopping kind of soothes me and makes me feel like I am doing something beneficial. In a way, I actually avoid thinking about that stressful moment.
But I end up wasting so much money and I don't even use most of the stuff that I bought.
How to stop bad habits?
1. Stop them from the root.
As I said my bad habit is to shop, so the root is the money. I made a budget and set a very limited amount of money to shop. So eventually I became well aware that I can't afford to shop because I am saving up for something else.
Before I did that budget, I used to blindly spend. I never actually cared to save up for anything. I always think "Oh, I would be able to manage it soon."
Only when I became mindful about my spending, I started being serious and took each spending into consideration whether it is vital or not.
2. Stop the distraction
Social media is made up of a world full of distractions and it is such eye candy to those who want to be spoilt. The number of ads I get for shopping is uncountable and the offers are so enticing that you will surely end up buying 4 mascaras from different brands at the same time when you don't even use one!
So what you do when this happens is STOP THE AD! There are buttons and settings around the ad where you can set it to " I don't want to see this". I did that to each and every ad I see about sales or offers until I got none.
The fewer sales I saw, the less I thought about SHOPPING.
3. Replace your bad habits
Some say you can't stop the bad habits, you can only replace it. Which makes a lot of sense. When you replace it, you will forget whatever you had before it. Instead of shopping, I can go to the gym or go for a walk instead. Exhaust me there, de-stress and completely distract myself.
I get an amazing body and I get to save so much money! What a winning situation right?

a number of an amount of 在 The amount of VS The number of, etc - English StackExchange 的推薦與評價
This question appears to arise from a comment I made in an earlier question. Op had written: The pie charts compare the amount of people ... ... <看更多>
a number of an amount of 在 Amount vs Number | Ask Linda! | English Grammar - YouTube 的推薦與評價

... <看更多>
a number of an amount of 在 常春藤英語- 【#多益小教室】 多益考試中描述數量的片語很多 的推薦與評價
A large number of students are against the controversial policy. 很多學生反對這具爭議性的政策。 . 2. a huge / large amount of + 不可數名詞很多 ... ... <看更多>